pathlib
— Object-oriented filesystem paths¶
Added in version 3.4.
Source code:Lib/pathlib.py
This module offers classes representing filesystem paths with semantics appropriate for different operating systems. Path classes are divided betweenpure paths,which provide purely computational operations without I/O, andconcrete paths,which inherit from pure paths but also provide I/O operations.
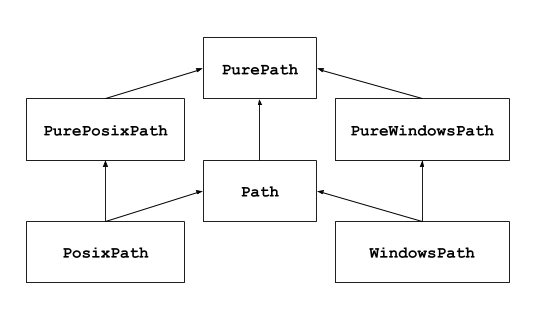
If you’ve never used this module before or just aren’t sure which class is
right for your task,Path
is most likely what you need. It instantiates
aconcrete pathfor the platform the code is running on.
Pure paths are useful in some special cases; for example:
If you want to manipulate Windows paths on a Unix machine (or vice versa). You cannot instantiate a
WindowsPath
when running on Unix, but you can instantiatePureWindowsPath
.You want to make sure that your code only manipulates paths without actually accessing the OS. In this case, instantiating one of the pure classes may be useful since those simply don’t have any OS-accessing operations.
See also
PEP 428:The pathlib module – object-oriented filesystem paths.
See also
For low-level path manipulation on strings, you can also use the
os.path
module.
Basic use¶
Importing the main class:
>>>frompathlibimportPath
Listing subdirectories:
>>>p=Path('.')
>>>[xforxinp.iterdir()ifx.is_dir()]
[PosixPath('.hg'), PosixPath('docs'), PosixPath('dist'),
PosixPath('__pycache__'), PosixPath('build')]
Listing Python source files in this directory tree:
>>>list(p.glob('**/*.py'))
[PosixPath('test_pathlib.py'), PosixPath('setup.py'),
PosixPath('pathlib.py'), PosixPath('docs/conf.py'),
PosixPath('build/lib/pathlib.py')]
Navigating inside a directory tree:
>>>p=Path('/etc')
>>>q=p/'init.d'/'reboot'
>>>q
PosixPath('/etc/init.d/reboot')
>>>q.resolve()
PosixPath('/etc/rc.d/init.d/halt')
Querying path properties:
>>>q.exists()
True
>>>q.is_dir()
False
Opening a file:
>>>withq.open()asf:f.readline()
...
'#!/bin/bash\n'
Pure paths¶
Pure path objects provide path-handling operations which don’t actually access a filesystem. There are three ways to access these classes, which we also callflavours:
- classpathlib.PurePath(*pathsegments)¶
A generic class that represents the system’s path flavour (instantiating it creates either a
PurePosixPath
or aPureWindowsPath
):>>>PurePath('setup.py')# Running on a Unix machine PurePosixPath('setup.py')
Each element ofpathsegmentscan be either a string representing a path segment, or an object implementing the
os.PathLike
interface where the__fspath__()
method returns a string, such as another path object:>>>PurePath('foo','some/path','bar') PurePosixPath('foo/some/path/bar') >>>PurePath(Path('foo'),Path('bar')) PurePosixPath('foo/bar')
Whenpathsegmentsis empty, the current directory is assumed:
>>>PurePath() PurePosixPath('.')
If a segment is an absolute path, all previous segments are ignored (like
os.path.join()
):>>>PurePath('/etc','/usr','lib64') PurePosixPath('/usr/lib64') >>>PureWindowsPath('c:/Windows','d:bar') PureWindowsPath('d:bar')
On Windows, the drive is not reset when a rooted relative path segment (e.g.,
r'\foo'
) is encountered:>>>PureWindowsPath('c:/Windows','/Program Files') PureWindowsPath('c:/Program Files')
Spurious slashes and single dots are collapsed, but double dots (
'..'
) and leading double slashes ('//'
) are not, since this would change the meaning of a path for various reasons (e.g. symbolic links, UNC paths):>>>PurePath('foo//bar') PurePosixPath('foo/bar') >>>PurePath('//foo/bar') PurePosixPath('//foo/bar') >>>PurePath('foo/./bar') PurePosixPath('foo/bar') >>>PurePath('foo/../bar') PurePosixPath('foo/../bar')
(a naïve approach would make
PurePosixPath('foo/../bar')
equivalent toPurePosixPath('bar')
,which is wrong iffoo
is a symbolic link to another directory)Pure path objects implement the
os.PathLike
interface, allowing them to be used anywhere the interface is accepted.Changed in version 3.6:Added support for the
os.PathLike
interface.
- classpathlib.PurePosixPath(*pathsegments)¶
A subclass of
PurePath
,this path flavour represents non-Windows filesystem paths:>>>PurePosixPath('/etc/hosts') PurePosixPath('/etc/hosts')
pathsegmentsis specified similarly to
PurePath
.
- classpathlib.PureWindowsPath(*pathsegments)¶
A subclass of
PurePath
,this path flavour represents Windows filesystem paths, includingUNC paths:>>>PureWindowsPath('c:/','Users','Ximénez') PureWindowsPath('c:/Users/Ximénez') >>>PureWindowsPath('//server/share/file') PureWindowsPath('//server/share/file')
pathsegmentsis specified similarly to
PurePath
.
Regardless of the system you’re running on, you can instantiate all of these classes, since they don’t provide any operation that does system calls.
General properties¶
Paths are immutable andhashable.Paths of a same flavour are comparable and orderable. These properties respect the flavour’s case-folding semantics:
>>>PurePosixPath('foo')==PurePosixPath('FOO')
False
>>>PureWindowsPath('foo')==PureWindowsPath('FOO')
True
>>>PureWindowsPath('FOO')in{PureWindowsPath('foo')}
True
>>>PureWindowsPath('C:')<PureWindowsPath('d:')
True
Paths of a different flavour compare unequal and cannot be ordered:
>>>PureWindowsPath('foo')==PurePosixPath('foo')
False
>>>PureWindowsPath('foo')<PurePosixPath('foo')
Traceback (most recent call last):
File"<stdin>",line1,in<module>
TypeError:'<' not supported between instances of 'PureWindowsPath' and 'PurePosixPath'
Operators¶
The slash operator helps create child paths, likeos.path.join()
.
If the argument is an absolute path, the previous path is ignored.
On Windows, the drive is not reset when the argument is a rooted
relative path (e.g.,r'\foo'
):
>>>p=PurePath('/etc')
>>>p
PurePosixPath('/etc')
>>>p/'init.d'/'apache2'
PurePosixPath('/etc/init.d/apache2')
>>>q=PurePath('bin')
>>>'/usr'/q
PurePosixPath('/usr/bin')
>>>p/'/an_absolute_path'
PurePosixPath('/an_absolute_path')
>>>PureWindowsPath('c:/Windows','/Program Files')
PureWindowsPath('c:/Program Files')
A path object can be used anywhere an object implementingos.PathLike
is accepted:
>>>importos
>>>p=PurePath('/etc')
>>>os.fspath(p)
'/etc'
The string representation of a path is the raw filesystem path itself (in native form, e.g. with backslashes under Windows), which you can pass to any function taking a file path as a string:
>>>p=PurePath('/etc')
>>>str(p)
'/etc'
>>>p=PureWindowsPath('c:/Program Files')
>>>str(p)
'c:\\Program Files'
Similarly, callingbytes
on a path gives the raw filesystem path as a
bytes object, as encoded byos.fsencode()
:
>>>bytes(p)
b'/etc'
Note
Callingbytes
is only recommended under Unix. Under Windows,
the unicode form is the canonical representation of filesystem paths.
Accessing individual parts¶
To access the individual “parts” (components) of a path, use the following property:
- PurePath.parts¶
A tuple giving access to the path’s various components:
>>>p=PurePath('/usr/bin/ Python 3') >>>p.parts ('/', 'usr', 'bin', ' Python 3') >>>p=PureWindowsPath('c:/Program Files/PSF') >>>p.parts ('c:\\', 'Program Files', 'PSF')
(note how the drive and local root are regrouped in a single part)
Methods and properties¶
Pure paths provide the following methods and properties:
- PurePath.drive¶
A string representing the drive letter or name, if any:
>>>PureWindowsPath('c:/Program Files/').drive 'c:' >>>PureWindowsPath('/Program Files/').drive '' >>>PurePosixPath('/etc').drive ''
UNC shares are also considered drives:
>>>PureWindowsPath('//host/share/foo.txt').drive '\\\\host\\share'
- PurePath.root¶
A string representing the (local or global) root, if any:
>>>PureWindowsPath('c:/Program Files/').root '\\' >>>PureWindowsPath('c:Program Files/').root '' >>>PurePosixPath('/etc').root '/'
UNC shares always have a root:
>>>PureWindowsPath('//host/share').root '\\'
If the path starts with more than two successive slashes,
PurePosixPath
collapses them:>>>PurePosixPath('//etc').root '//' >>>PurePosixPath('///etc').root '/' >>>PurePosixPath('////etc').root '/'
Note
This behavior conforms toThe Open Group Base Specifications Issue 6, paragraph4.11 Pathname Resolution:
“A pathname that begins with two successive slashes may be interpreted in an implementation-defined manner, although more than two leading slashes shall be treated as a single slash.”
- PurePath.anchor¶
The concatenation of the drive and root:
>>>PureWindowsPath('c:/Program Files/').anchor 'c:\\' >>>PureWindowsPath('c:Program Files/').anchor 'c:' >>>PurePosixPath('/etc').anchor '/' >>>PureWindowsPath('//host/share').anchor '\\\\host\\share\\'
- PurePath.parents¶
An immutable sequence providing access to the logical ancestors of the path:
>>>p=PureWindowsPath('c:/foo/bar/setup.py') >>>p.parents[0] PureWindowsPath('c:/foo/bar') >>>p.parents[1] PureWindowsPath('c:/foo') >>>p.parents[2] PureWindowsPath('c:/')
Changed in version 3.10:The parents sequence now supportsslicesand negative index values.
- PurePath.parent¶
The logical parent of the path:
>>>p=PurePosixPath('/a/b/c/d') >>>p.parent PurePosixPath('/a/b/c')
You cannot go past an anchor, or empty path:
>>>p=PurePosixPath('/') >>>p.parent PurePosixPath('/') >>>p=PurePosixPath('.') >>>p.parent PurePosixPath('.')
Note
This is a purely lexical operation, hence the following behaviour:
>>>p=PurePosixPath('foo/..') >>>p.parent PurePosixPath('foo')
If you want to walk an arbitrary filesystem path upwards, it is recommended to first call
Path.resolve()
so as to resolve symlinks and eliminate".."
components.
- PurePath.name¶
A string representing the final path component, excluding the drive and root, if any:
>>>PurePosixPath('my/library/setup.py').name 'setup.py'
UNC drive names are not considered:
>>>PureWindowsPath('//some/share/setup.py').name 'setup.py' >>>PureWindowsPath('//some/share').name ''
- PurePath.suffix¶
The file extension of the final component, if any:
>>>PurePosixPath('my/library/setup.py').suffix '.py' >>>PurePosixPath('my/library.tar.gz').suffix '.gz' >>>PurePosixPath('my/library').suffix ''
- PurePath.suffixes¶
A list of the path’s file extensions:
>>>PurePosixPath('my/library.tar.gar').suffixes ['.tar', '.gar'] >>>PurePosixPath('my/library.tar.gz').suffixes ['.tar', '.gz'] >>>PurePosixPath('my/library').suffixes []
- PurePath.stem¶
The final path component, without its suffix:
>>>PurePosixPath('my/library.tar.gz').stem 'library.tar' >>>PurePosixPath('my/library.tar').stem 'library' >>>PurePosixPath('my/library').stem 'library'
- PurePath.as_posix()¶
Return a string representation of the path with forward slashes (
/
):>>>p=PureWindowsPath('c:\\windows') >>>str(p) 'c:\\windows' >>>p.as_posix() 'c:/windows'
- PurePath.as_uri()¶
Represent the path as a
file
URI.ValueError
is raised if the path isn’t absolute.>>>p=PurePosixPath('/etc/passwd') >>>p.as_uri() 'file:///etc/passwd' >>>p=PureWindowsPath('c:/Windows') >>>p.as_uri() 'file:///c:/Windows'
- PurePath.is_absolute()¶
Return whether the path is absolute or not. A path is considered absolute if it has both a root and (if the flavour allows) a drive:
>>>PurePosixPath('/a/b').is_absolute() True >>>PurePosixPath('a/b').is_absolute() False >>>PureWindowsPath('c:/a/b').is_absolute() True >>>PureWindowsPath('/a/b').is_absolute() False >>>PureWindowsPath('c:').is_absolute() False >>>PureWindowsPath('//some/share').is_absolute() True
- PurePath.is_relative_to(other)¶
Return whether or not this path is relative to theotherpath.
>>>p=PurePath('/etc/passwd') >>>p.is_relative_to('/etc') True >>>p.is_relative_to('/usr') False
This method is string-based; it neither accesses the filesystem nor treats “
..
”segments specially. The following code is equivalent:>>>u=PurePath('/usr') >>>u==poruinp.parents False
Added in version 3.9.
Deprecated since version 3.12, will be removed in version 3.14:Passing additional arguments is deprecated; if supplied, they are joined withother.
- PurePath.is_reserved()¶
With
PureWindowsPath
,returnTrue
if the path is considered reserved under Windows,False
otherwise. WithPurePosixPath
,False
is always returned.>>>PureWindowsPath('nul').is_reserved() True >>>PurePosixPath('nul').is_reserved() False
File system calls on reserved paths can fail mysteriously or have unintended effects.
- PurePath.joinpath(*pathsegments)¶
Calling this method is equivalent to combining the path with each of the givenpathsegmentsin turn:
>>>PurePosixPath('/etc').joinpath('passwd') PurePosixPath('/etc/passwd') >>>PurePosixPath('/etc').joinpath(PurePosixPath('passwd')) PurePosixPath('/etc/passwd') >>>PurePosixPath('/etc').joinpath('init.d','apache2') PurePosixPath('/etc/init.d/apache2') >>>PureWindowsPath('c:').joinpath('/Program Files') PureWindowsPath('c:/Program Files')
- PurePath.match(pattern,*,case_sensitive=None)¶
Match this path against the provided glob-style pattern. Return
True
if matching is successful,False
otherwise.Ifpatternis relative, the path can be either relative or absolute, and matching is done from the right:
>>>PurePath('a/b.py').match('*.py') True >>>PurePath('/a/b/c.py').match('b/*.py') True >>>PurePath('/a/b/c.py').match('a/*.py') False
Ifpatternis absolute, the path must be absolute, and the whole path must match:
>>>PurePath('/a.py').match('/*.py') True >>>PurePath('a/b.py').match('/*.py') False
Thepatternmay be another path object; this speeds up matching the same pattern against multiple files:
>>>pattern=PurePath('*.py') >>>PurePath('a/b.py').match(pattern) True
Note
The recursive wildcard “
**
”isn’t supported by this method (it acts like non-recursive “*
”.)Changed in version 3.12:Accepts an object implementing the
os.PathLike
interface.As with other methods, case-sensitivity follows platform defaults:
>>>PurePosixPath('b.py').match('*.PY') False >>>PureWindowsPath('b.py').match('*.PY') True
Setcase_sensitiveto
True
orFalse
to override this behaviour.Changed in version 3.12:Thecase_sensitiveparameter was added.
- PurePath.relative_to(other,walk_up=False)¶
Compute a version of this path relative to the path represented by other.If it’s impossible,
ValueError
is raised:>>>p=PurePosixPath('/etc/passwd') >>>p.relative_to('/') PurePosixPath('etc/passwd') >>>p.relative_to('/etc') PurePosixPath('passwd') >>>p.relative_to('/usr') Traceback (most recent call last): File"<stdin>",line1,in<module> File"pathlib.py",line941,inrelative_to raiseValueError(error_message.format(str(self),str(formatted))) ValueError:'/etc/passwd' is not in the subpath of '/usr' OR one path is relative and the other is absolute.
Whenwalk_upis false (the default), the path must start withother. When the argument is true,
..
entries may be added to form the relative path. In all other cases, such as the paths referencing different drives,ValueError
is raised.:>>>p.relative_to('/usr',walk_up=True) PurePosixPath('../etc/passwd') >>>p.relative_to('foo',walk_up=True) Traceback (most recent call last): File"<stdin>",line1,in<module> File"pathlib.py",line941,inrelative_to raiseValueError(error_message.format(str(self),str(formatted))) ValueError:'/etc/passwd' is not on the same drive as 'foo' OR one path is relative and the other is absolute.
Warning
This function is part of
PurePath
and works with strings. It does not check or access the underlying file structure. This can impact thewalk_upoption as it assumes that no symlinks are present in the path; callresolve()
first if necessary to resolve symlinks.Changed in version 3.12:Thewalk_upparameter was added (old behavior is the same as
walk_up=False
).Deprecated since version 3.12, will be removed in version 3.14:Passing additional positional arguments is deprecated; if supplied, they are joined withother.
- PurePath.with_name(name)¶
Return a new path with the
name
changed. If the original path doesn’t have a name, ValueError is raised:>>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>>p.with_name('setup.py') PureWindowsPath('c:/Downloads/setup.py') >>>p=PureWindowsPath('c:/') >>>p.with_name('setup.py') Traceback (most recent call last): File"<stdin>",line1,in<module> File"/home/antoine/c Python /default/Lib/pathlib.py",line751,inwith_name raiseValueError("%rhas an empty name "%(self,)) ValueError:PureWindowsPath('c:/') has an empty name
- PurePath.with_stem(stem)¶
Return a new path with the
stem
changed. If the original path doesn’t have a name, ValueError is raised:>>>p=PureWindowsPath('c:/Downloads/draft.txt') >>>p.with_stem('final') PureWindowsPath('c:/Downloads/final.txt') >>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>>p.with_stem('lib') PureWindowsPath('c:/Downloads/lib.gz') >>>p=PureWindowsPath('c:/') >>>p.with_stem('') Traceback (most recent call last): File"<stdin>",line1,in<module> File"/home/antoine/c Python /default/Lib/pathlib.py",line861,inwith_stem returnself.with_name(stem+self.suffix) File"/home/antoine/c Python /default/Lib/pathlib.py",line851,inwith_name raiseValueError("%rhas an empty name "%(self,)) ValueError:PureWindowsPath('c:/') has an empty name
Added in version 3.9.
- PurePath.with_suffix(suffix)¶
Return a new path with the
suffix
changed. If the original path doesn’t have a suffix, the newsuffixis appended instead. If the suffixis an empty string, the original suffix is removed:>>>p=PureWindowsPath('c:/Downloads/pathlib.tar.gz') >>>p.with_suffix('.bz2') PureWindowsPath('c:/Downloads/pathlib.tar.bz2') >>>p=PureWindowsPath('README') >>>p.with_suffix('.txt') PureWindowsPath('README.txt') >>>p=PureWindowsPath('README.txt') >>>p.with_suffix('') PureWindowsPath('README')
- PurePath.with_segments(*pathsegments)¶
Create a new path object of the same type by combining the given pathsegments.This method is called whenever a derivative path is created, such as from
parent
andrelative_to()
.Subclasses may override this method to pass information to derivative paths, for example:frompathlibimportPurePosixPath classMyPath(PurePosixPath): def__init__(self,*pathsegments,session_id): super().__init__(*pathsegments) self.session_id=session_id defwith_segments(self,*pathsegments): returntype(self)(*pathsegments,session_id=self.session_id) etc=MyPath('/etc',session_id=42) hosts=etc/'hosts' print(hosts.session_id)# 42
Added in version 3.12.
Concrete paths¶
Concrete paths are subclasses of the pure path classes. In addition to operations provided by the latter, they also provide methods to do system calls on path objects. There are three ways to instantiate concrete paths:
- classpathlib.Path(*pathsegments)¶
A subclass of
PurePath
,this class represents concrete paths of the system’s path flavour (instantiating it creates either aPosixPath
or aWindowsPath
):>>>Path('setup.py') PosixPath('setup.py')
pathsegmentsis specified similarly to
PurePath
.
- classpathlib.PosixPath(*pathsegments)¶
A subclass of
Path
andPurePosixPath
,this class represents concrete non-Windows filesystem paths:>>>PosixPath('/etc/hosts') PosixPath('/etc/hosts')
pathsegmentsis specified similarly to
PurePath
.
- classpathlib.WindowsPath(*pathsegments)¶
A subclass of
Path
andPureWindowsPath
,this class represents concrete Windows filesystem paths:>>>WindowsPath('c:/','Users','Ximénez') WindowsPath('c:/Users/Ximénez')
pathsegmentsis specified similarly to
PurePath
.
You can only instantiate the class flavour that corresponds to your system (allowing system calls on non-compatible path flavours could lead to bugs or failures in your application):
>>>importos
>>>os.name
'posix'
>>>Path('setup.py')
PosixPath('setup.py')
>>>PosixPath('setup.py')
PosixPath('setup.py')
>>>WindowsPath('setup.py')
Traceback (most recent call last):
File"<stdin>",line1,in<module>
File"pathlib.py",line798,in__new__
%(cls.__name__,))
NotImplementedError:cannot instantiate 'WindowsPath' on your system
Some concrete path methods can raise anOSError
if a system call fails
(for example because the path doesn’t exist).
Expanding and resolving paths¶
- classmethodPath.home()¶
Return a new path object representing the user’s home directory (as returned by
os.path.expanduser()
with~
construct). If the home directory can’t be resolved,RuntimeError
is raised.>>>Path.home() PosixPath('/home/antoine')
Added in version 3.5.
- Path.expanduser()¶
Return a new path with expanded
~
and~user
constructs, as returned byos.path.expanduser()
.If a home directory can’t be resolved,RuntimeError
is raised.>>>p=PosixPath('~/films/Monty Python') >>>p.expanduser() PosixPath('/home/eric/films/Monty Python')
Added in version 3.5.
- classmethodPath.cwd()¶
Return a new path object representing the current directory (as returned by
os.getcwd()
):>>>Path.cwd() PosixPath('/home/antoine/pathlib')
- Path.absolute()¶
Make the path absolute, without normalization or resolving symlinks. Returns a new path object:
>>>p=Path('tests') >>>p PosixPath('tests') >>>p.absolute() PosixPath('/home/antoine/pathlib/tests')
- Path.resolve(strict=False)¶
Make the path absolute, resolving any symlinks. A new path object is returned:
>>>p=Path() >>>p PosixPath('.') >>>p.resolve() PosixPath('/home/antoine/pathlib')
“
..
”components are also eliminated (this is the only method to do so):>>>p=Path('docs/../setup.py') >>>p.resolve() PosixPath('/home/antoine/pathlib/setup.py')
If the path doesn’t exist andstrictis
True
,FileNotFoundError
is raised. IfstrictisFalse
,the path is resolved as far as possible and any remainder is appended without checking whether it exists. If an infinite loop is encountered along the resolution path,RuntimeError
is raised.Changed in version 3.6:Thestrictparameter was added (pre-3.6 behavior is strict).
- Path.readlink()¶
Return the path to which the symbolic link points (as returned by
os.readlink()
):>>>p=Path('mylink') >>>p.symlink_to('setup.py') >>>p.readlink() PosixPath('setup.py')
Added in version 3.9.
Querying file type and status¶
Changed in version 3.8:exists()
,is_dir()
,is_file()
,
is_mount()
,is_symlink()
,
is_block_device()
,is_char_device()
,
is_fifo()
,is_socket()
now returnFalse
instead of raising an exception for paths that contain characters
unrepresentable at the OS level.
- Path.stat(*,follow_symlinks=True)¶
Return an
os.stat_result
object containing information about this path, likeos.stat()
. The result is looked up at each call to this method.This method normally follows symlinks; to stat a symlink add the argument
follow_symlinks=False
,or uselstat()
.>>>p=Path('setup.py') >>>p.stat().st_size 956 >>>p.stat().st_mtime 1327883547.852554
Changed in version 3.10:Thefollow_symlinksparameter was added.
- Path.lstat()¶
Like
Path.stat()
but, if the path points to a symbolic link, return the symbolic link’s information rather than its target’s.
- Path.exists(*,follow_symlinks=True)¶
Return
True
if the path points to an existing file or directory.This method normally follows symlinks; to check if a symlink exists, add the argument
follow_symlinks=False
.>>>Path('.').exists() True >>>Path('setup.py').exists() True >>>Path('/etc').exists() True >>>Path('nonexistentfile').exists() False
Changed in version 3.12:Thefollow_symlinksparameter was added.
- Path.is_file()¶
Return
True
if the path points to a regular file (or a symbolic link pointing to a regular file),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_dir()¶
Return
True
if the path points to a directory (or a symbolic link pointing to a directory),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_symlink()¶
Return
True
if the path points to a symbolic link,False
otherwise.False
is also returned if the path doesn’t exist; other errors (such as permission errors) are propagated.
- Path.is_junction()¶
Return
True
if the path points to a junction, andFalse
for any other type of file. Currently only Windows supports junctions.Added in version 3.12.
- Path.is_mount()¶
Return
True
if the path is amount point:a point in a file system where a different file system has been mounted. On POSIX, the function checks whetherpath’s parent,path/..
,is on a different device thanpath,or whetherpath/..
andpathpoint to the same i-node on the same device — this should detect mount points for all Unix and POSIX variants. On Windows, a mount point is considered to be a drive letter root (e.g.c:\
), a UNC share (e.g.\\server\share
), or a mounted filesystem directory.Added in version 3.7.
Changed in version 3.12:Windows support was added.
- Path.is_socket()¶
Return
True
if the path points to a Unix socket (or a symbolic link pointing to a Unix socket),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_fifo()¶
Return
True
if the path points to a FIFO (or a symbolic link pointing to a FIFO),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_block_device()¶
Return
True
if the path points to a block device (or a symbolic link pointing to a block device),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.is_char_device()¶
Return
True
if the path points to a character device (or a symbolic link pointing to a character device),False
if it points to another kind of file.False
is also returned if the path doesn’t exist or is a broken symlink; other errors (such as permission errors) are propagated.
- Path.samefile(other_path)¶
Return whether this path points to the same file asother_path,which can be either a Path object, or a string. The semantics are similar to
os.path.samefile()
andos.path.samestat()
.An
OSError
can be raised if either file cannot be accessed for some reason.>>>p=Path('spam') >>>q=Path('eggs') >>>p.samefile(q) False >>>p.samefile('spam') True
Added in version 3.5.
Reading and writing files¶
- Path.open(mode='r',buffering=-1,encoding=None,errors=None,newline=None)¶
Open the file pointed to by the path, like the built-in
open()
function does:>>>p=Path('setup.py') >>>withp.open()asf: ...f.readline() ... '#!/usr/bin/env Python 3\n'
- Path.read_text(encoding=None,errors=None)¶
Return the decoded contents of the pointed-to file as a string:
>>>p=Path('my_text_file') >>>p.write_text('Text file contents') 18 >>>p.read_text() 'Text file contents'
The file is opened and then closed. The optional parameters have the same meaning as in
open()
.Added in version 3.5.
- Path.read_bytes()¶
Return the binary contents of the pointed-to file as a bytes object:
>>>p=Path('my_binary_file') >>>p.write_bytes(b'Binary file contents') 20 >>>p.read_bytes() b'Binary file contents'
Added in version 3.5.
- Path.write_text(data,encoding=None,errors=None,newline=None)¶
Open the file pointed to in text mode, writedatato it, and close the file:
>>>p=Path('my_text_file') >>>p.write_text('Text file contents') 18 >>>p.read_text() 'Text file contents'
An existing file of the same name is overwritten. The optional parameters have the same meaning as in
open()
.Added in version 3.5.
Changed in version 3.10:Thenewlineparameter was added.
- Path.write_bytes(data)¶
Open the file pointed to in bytes mode, writedatato it, and close the file:
>>>p=Path('my_binary_file') >>>p.write_bytes(b'Binary file contents') 20 >>>p.read_bytes() b'Binary file contents'
An existing file of the same name is overwritten.
Added in version 3.5.
Reading directories¶
- Path.iterdir()¶
When the path points to a directory, yield path objects of the directory contents:
>>>p=Path('docs') >>>forchildinp.iterdir():child ... PosixPath('docs/conf.py') PosixPath('docs/_templates') PosixPath('docs/make.bat') PosixPath('docs/index.rst') PosixPath('docs/_build') PosixPath('docs/_static') PosixPath('docs/Makefile')
The children are yielded in arbitrary order, and the special entries
'.'
and'..'
are not included. If a file is removed from or added to the directory after creating the iterator, it is unspecified whether a path object for that file is included.If the path is not a directory or otherwise inaccessible,
OSError
is raised.
- Path.glob(pattern,*,case_sensitive=None)¶
Glob the given relativepatternin the directory represented by this path, yielding all matching files (of any kind):
>>>sorted(Path('.').glob('*.py')) [PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')] >>>sorted(Path('.').glob('*/*.py')) [PosixPath('docs/conf.py')]
Patterns are the same as for
fnmatch
,with the addition of “**
” which means “this directory and all subdirectories, recursively”. In other words, it enables recursive globbing:>>>sorted(Path('.').glob('**/*.py')) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
This method calls
Path.is_dir()
on the top-level directory and propagates anyOSError
exception that is raised. SubsequentOSError
exceptions from scanning directories are suppressed.By default, or when thecase_sensitivekeyword-only argument is set to
None
,this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Setcase_sensitivetoTrue
orFalse
to override this behaviour.Note
Using the “
**
”pattern in large directory trees may consume an inordinate amount of time.Raises anauditing event
pathlib.Path.glob
with argumentsself
,pattern
.Changed in version 3.11:Return only directories ifpatternends with a pathname components separator (
sep
oraltsep
).Changed in version 3.12:Thecase_sensitiveparameter was added.
- Path.rglob(pattern,*,case_sensitive=None)¶
Glob the given relativepatternrecursively. This is like calling
Path.glob()
with “**/
”added in front of thepattern,where patternsare the same as forfnmatch
:>>>sorted(Path().rglob("*.py")) [PosixPath('build/lib/pathlib.py'), PosixPath('docs/conf.py'), PosixPath('pathlib.py'), PosixPath('setup.py'), PosixPath('test_pathlib.py')]
By default, or when thecase_sensitivekeyword-only argument is set to
None
,this method matches paths using platform-specific casing rules: typically, case-sensitive on POSIX, and case-insensitive on Windows. Setcase_sensitivetoTrue
orFalse
to override this behaviour.Raises anauditing event
pathlib.Path.rglob
with argumentsself
,pattern
.Changed in version 3.11:Return only directories ifpatternends with a pathname components separator (
sep
oraltsep
).Changed in version 3.12:Thecase_sensitiveparameter was added.
- Path.walk(top_down=True,on_error=None,follow_symlinks=False)¶
Generate the file names in a directory tree by walking the tree either top-down or bottom-up.
For each directory in the directory tree rooted atself(including selfbut excluding ‘.’ and ‘..’), the method yields a 3-tuple of
(dirpath,dirnames,filenames)
.dirpathis a
Path
to the directory currently being walked, dirnamesis a list of strings for the names of subdirectories indirpath (excluding'.'
and'..'
), andfilenamesis a list of strings for the names of the non-directory files indirpath.To get a full path (which begins withself) to a file or directory indirpath,dodirpath/name
.Whether or not the lists are sorted is file system-dependent.If the optional argumenttop_downis true (which is the default), the triple for a directory is generated before the triples for any of its subdirectories (directories are walked top-down). Iftop_downis false, the triple for a directory is generated after the triples for all of its subdirectories (directories are walked bottom-up). No matter the value oftop_down,the list of subdirectories is retrieved before the triples for the directory and its subdirectories are walked.
Whentop_downis true, the caller can modify thedirnameslist in-place (for example, using
del
or slice assignment), andPath.walk()
will only recurse into the subdirectories whose names remain indirnames. This can be used to prune the search, or to impose a specific order of visiting, or even to informPath.walk()
about directories the caller creates or renames before it resumesPath.walk()
again. Modifyingdirnameswhen top_downis false has no effect on the behavior ofPath.walk()
since the directories indirnameshave already been generated by the timedirnames is yielded to the caller.By default, errors from
os.scandir()
are ignored. If the optional argumenton_erroris specified, it should be a callable; it will be called with one argument, anOSError
instance. The callable can handle the error to continue the walk or re-raise it to stop the walk. Note that the filename is available as thefilename
attribute of the exception object.By default,
Path.walk()
does not follow symbolic links, and instead adds them to thefilenameslist. Setfollow_symlinksto true to resolve symlinks and place them indirnamesandfilenamesas appropriate for their targets, and consequently visit directories pointed to by symlinks (where supported).Note
Be aware that settingfollow_symlinksto true can lead to infinite recursion if a link points to a parent directory of itself.
Path.walk()
does not keep track of the directories it has already visited.Note
Path.walk()
assumes the directories it walks are not modified during execution. For example, if a directory fromdirnameshas been replaced with a symlink andfollow_symlinksis false,Path.walk()
will still try to descend into it. To prevent such behavior, remove directories fromdirnamesas appropriate.Note
Unlike
os.walk()
,Path.walk()
lists symlinks to directories in filenamesiffollow_symlinksis false.This example displays the number of bytes used by all files in each directory, while ignoring
__pycache__
directories:frompathlibimportPath forroot,dirs,filesinPath("c Python /Lib/concurrent").walk(on_error=print): print( root, "consumes", sum((root/file).stat().st_sizeforfileinfiles), "bytes in", len(files), "non-directory files" ) if'__pycache__'indirs: dirs.remove('__pycache__')
This next example is a simple implementation of
shutil.rmtree()
. Walking the tree bottom-up is essential asrmdir()
doesn’t allow deleting a directory before it is empty:# Delete everything reachable from the directory "top". # CAUTION: This is dangerous! For example, if top == Path('/'), # it could delete all of your files. forroot,dirs,filesintop.walk(top_down=False): fornameinfiles: (root/name).unlink() fornameindirs: (root/name).rmdir()
Added in version 3.12.
Creating files and directories¶
- Path.touch(mode=0o666,exist_ok=True)¶
Create a file at this given path. Ifmodeis given, it is combined with the process’s
umask
value to determine the file mode and access flags. If the file already exists, the function succeeds whenexist_ok is true (and its modification time is updated to the current time), otherwiseFileExistsError
is raised.See also
The
open()
,write_text()
andwrite_bytes()
methods are often used to create files.
- Path.mkdir(mode=0o777,parents=False,exist_ok=False)¶
Create a new directory at this given path. Ifmodeis given, it is combined with the process’s
umask
value to determine the file mode and access flags. If the path already exists,FileExistsError
is raised.Ifparentsis true, any missing parents of this path are created as needed; they are created with the default permissions without taking modeinto account (mimicking the POSIX
mkdir-p
command).Ifparentsis false (the default), a missing parent raises
FileNotFoundError
.Ifexist_okis false (the default),
FileExistsError
is raised if the target directory already exists.Ifexist_okis true,
FileExistsError
will not be raised unless the given path already exists in the file system and is not a directory (same behavior as the POSIXmkdir-p
command).Changed in version 3.5:Theexist_okparameter was added.
- Path.symlink_to(target,target_is_directory=False)¶
Make this path a symbolic link pointing totarget.
On Windows, a symlink represents either a file or a directory, and does not morph to the target dynamically. If the target is present, the type of the symlink will be created to match. Otherwise, the symlink will be created as a directory iftarget_is_directoryis true or a file symlink (the default) otherwise. On non-Windows platforms,target_is_directoryis ignored.
>>>p=Path('mylink') >>>p.symlink_to('setup.py') >>>p.resolve() PosixPath('/home/antoine/pathlib/setup.py') >>>p.stat().st_size 956 >>>p.lstat().st_size 8
Note
The order of arguments (link, target) is the reverse of
os.symlink()
’s.
Renaming and deleting¶
- Path.rename(target)¶
Rename this file or directory to the giventarget,and return a new
Path
instance pointing totarget.On Unix, iftargetexists and is a file, it will be replaced silently if the user has permission. On Windows, iftargetexists,FileExistsError
will be raised. targetcan be either a string or another path object:>>>p=Path('foo') >>>p.open('w').write('some text') 9 >>>target=Path('bar') >>>p.rename(target) PosixPath('bar') >>>target.open().read() 'some text'
The target path may be absolute or relative. Relative paths are interpreted relative to the current working directory,notthe directory of the
Path
object.It is implemented in terms of
os.rename()
and gives the same guarantees.Changed in version 3.8:Added return value, return the new
Path
instance.
- Path.replace(target)¶
Rename this file or directory to the giventarget,and return a new
Path
instance pointing totarget.Iftargetpoints to an existing file or empty directory, it will be unconditionally replaced.The target path may be absolute or relative. Relative paths are interpreted relative to the current working directory,notthe directory of the
Path
object.Changed in version 3.8:Added return value, return the new
Path
instance.
- Path.unlink(missing_ok=False)¶
Remove this file or symbolic link. If the path points to a directory, use
Path.rmdir()
instead.Ifmissing_okis false (the default),
FileNotFoundError
is raised if the path does not exist.Ifmissing_okis true,
FileNotFoundError
exceptions will be ignored (same behavior as the POSIXrm-f
command).Changed in version 3.8:Themissing_okparameter was added.
- Path.rmdir()¶
Remove this directory. The directory must be empty.
Permissions and ownership¶
- Path.owner()¶
Return the name of the user owning the file.
KeyError
is raised if the file’s user identifier (UID) isn’t found in the system database.
- Path.group()¶
Return the name of the group owning the file.
KeyError
is raised if the file’s group identifier (GID) isn’t found in the system database.
- Path.chmod(mode,*,follow_symlinks=True)¶
Change the file mode and permissions, like
os.chmod()
.This method normally follows symlinks. Some Unix flavours support changing permissions on the symlink itself; on these platforms you may add the argument
follow_symlinks=False
,or uselchmod()
.>>>p=Path('setup.py') >>>p.stat().st_mode 33277 >>>p.chmod(0o444) >>>p.stat().st_mode 33060
Changed in version 3.10:Thefollow_symlinksparameter was added.
- Path.lchmod(mode)¶
Like
Path.chmod()
but, if the path points to a symbolic link, the symbolic link’s mode is changed rather than its target’s.
Correspondence to tools in theos
module¶
Below is a table mapping variousos
functions to their corresponding
PurePath
/Path
equivalent.
Footnotes