/*
* copyright (c) 2001 Fabrice Bellard
*
* This file is part of FFmpeg.
*
* FFmpeg is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* FFmpeg is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with FFmpeg; if not, write to the Free Software
* Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
*/
#ifndef AVCODEC_AVCODEC_H
#define AVCODEC_AVCODEC_H
/**
* @file
* @ingroup libavc
* Libavcodec external API header
*/
#include <errno.h>
#include "libavutil/samplefmt.h"
#include "libavutil/attributes.h"
#include "libavutil/avutil.h"
#include "libavutil/buffer.h"
#include "libavutil/cpu.h"
#include "libavutil/channel_layout.h"
#include "libavutil/dict.h"
#include "libavutil/frame.h"
#include "libavutil/log.h"
#include "libavutil/pixfmt.h"
#include "libavutil/rational.h"
#include "version.h"
/**
* @defgroup libavc libavcodec
* Encoding/Decoding Library
*
* @{
*
* @defgroup lavc_decoding Decoding
* @{
* @}
*
* @defgroup lavc_encoding Encoding
* @{
* @}
*
* @defgroup lavc_codec Codecs
* @{
* @defgroup lavc_codec_native Native Codecs
* @{
* @}
* @defgroup lavc_codec_wrappers External library wrappers
* @{
* @}
* @defgroup lavc_codec_hwaccel Hardware Accelerators bridge
* @{
* @}
* @}
* @defgroup lavc_internal Internal
* @{
* @}
* @}
*/
/**
* @ingroup libavc
* @defgroup lavc_encdec send/receive encoding and decoding API overview
* @{
*
* The avcodec_send_packet()/avcodec_receive_frame()/avcodec_send_frame()/
* avcodec_receive_packet() functions provide an encode/decode API, which
* decouples input and output.
*
* The API is very similar for encoding/decoding and audio/video, and works as
* follows:
* - Set up and open the AVCodecContext as usual.
* - Send valid input:
* - For decoding, call avcodec_send_packet() to give the decoder raw
* compressed data in an AVPacket.
* - For encoding, call avcodec_send_frame() to give the encoder an AVFrame
* containing uncompressed audio or video.
* In both cases, it is recommended that AVPackets and AVFrames are
* refcounted, or libavcodec might have to copy the input data. (libavformat
* always returns refcounted AVPackets, and av_frame_get_buffer() allocates
* refcounted AVFrames.)
* - Receive output in a loop. Periodically call one of the avcodec_receive_*()
* functions and process their output:
* - For decoding, call avcodec_receive_frame(). On success, it will return
* an AVFrame containing uncompressed audio or video data.
* - For encoding, call avcodec_receive_packet(). On success, it will return
* an AVPacket with a compressed frame.
* Repeat this call until it returns AVERROR(EAGAIN) or an error. The
* AVERROR(EAGAIN) return value means that new input data is required to
* return new output. In this case, continue with sending input. For each
* input frame/packet, the codec will typically return 1 output frame/packet,
* but it can also be 0 or more than 1.
*
* At the beginning of decoding or encoding, the codec might accept multiple
* input frames/packets without returning a frame, until its internal buffers
* are filled. This situation is handled transparently if you follow the steps
* outlined above.
*
* In theory, sending input can result in EAGAIN - this should happen only if
* not all output was received. You can use this to structure alternative decode
* or encode loops other than the one suggested above. For example, you could
* try sending new input on each iteration, and try to receive output if that
* returns EAGAIN.
*
* End of stream situations. These require "flushing" (aka draining) the codec,
* as the codec might buffer multiple frames or packets internally for
* performance or out of necessity (consider B-frames).
* This is handled as follows:
* - Instead of valid input, send NULL to the avcodec_send_packet() (decoding)
* or avcodec_send_frame() (encoding) functions. This will enter draining
* mode.
* - Call avcodec_receive_frame() (decoding) or avcodec_receive_packet()
* (encoding) in a loop until AVERROR_EOF is returned. The functions will
* not return AVERROR(EAGAIN), unless you forgot to enter draining mode.
* - Before decoding can be resumed again, the codec has to be reset with
* avcodec_flush_buffers().
*
* Using the API as outlined above is highly recommended. But it is also
* possible to call functions outside of this rigid schema. For example, you can
* call avcodec_send_packet() repeatedly without calling
* avcodec_receive_frame(). In this case, avcodec_send_packet() will succeed
* until the codec's internal buffer has been filled up (which is typically of
* size 1 per output frame, after initial input), and then reject input with
* AVERROR(EAGAIN). Once it starts rejecting input, you have no choice but to
* read at least some output.
*
* Not all codecs will follow a rigid and predictable dataflow; the only
* guarantee is that an AVERROR(EAGAIN) return value on a send/receive call on
* one end implies that a receive/send call on the other end will succeed, or
* at least will not fail with AVERROR(EAGAIN). In general, no codec will
* permit unlimited buffering of input or output.
*
* This API replaces the following legacy functions:
* - avcodec_decode_video2() and avcodec_decode_audio4():
* Use avcodec_send_packet() to feed input to the decoder, then use
* avcodec_receive_frame() to receive decoded frames after each packet.
* Unlike with the old video decoding API, multiple frames might result from
* a packet. For audio, splitting the input packet into frames by partially
* decoding packets becomes transparent to the API user. You never need to
* feed an AVPacket to the API twice (unless it is rejected with AVERROR(EAGAIN) - then
* no data was read from the packet).
* Additionally, sending a flush/draining packet is required only once.
* - avcodec_encode_video2()/avcodec_encode_audio2():
* Use avcodec_send_frame() to feed input to the encoder, then use
* avcodec_receive_packet() to receive encoded packets.
* Providing user-allocated buffers for avcodec_receive_packet() is not
* possible.
* - The new API does not handle subtitles yet.
*
* Mixing new and old function calls on the same AVCodecContext is not allowed,
* and will result in undefined behavior.
*
* Some codecs might require using the new API; using the old API will return
* an error when calling it. All codecs support the new API.
*
* A codec is not allowed to return AVERROR(EAGAIN) for both sending and receiving. This
* would be an invalid state, which could put the codec user into an endless
* loop. The API has no concept of time either: it cannot happen that trying to
* do avcodec_send_packet() results in AVERROR(EAGAIN), but a repeated call 1 second
* later accepts the packet (with no other receive/flush API calls involved).
* The API is a strict state machine, and the passage of time is not supposed
* to influence it. Some timing-dependent behavior might still be deemed
* acceptable in certain cases. But it must never result in both send/receive
* returning EAGAIN at the same time at any point. It must also absolutely be
* avoided that the current state is "unstable" and can "flip-flop" between
* the send/receive APIs allowing progress. For example, it's not allowed that
* the codec randomly decides that it actually wants to consume a packet now
* instead of returning a frame, after it just returned AVERROR(EAG

vanjoge
- 粉丝: 60
- 资源: 1
最新资源
- 基于多目标优化的物流与冷链车辆路径规划与优化研究:考虑时间窗、成本与碳排放的遗传算法改进及多配送中心策略,基于多目标优化的物流与冷链车辆路径规划与优化算法研究,matlab车辆路径优化vrp,车辆路径
- 零基础入门转录组下游分析-机器学习算法之boruta(筛选特征基因)教程配套资源
- 基于Comsol的三维晶体轴压模拟:应用三维Voronoi算法与泰森多边形晶格分布的模型研究,基于三维Voronoi算法的comsol模型中三维晶体轴压模拟与泰森多边形晶格分布研究,comsol三维晶
- 双极性SPWM单相全桥逆变电路仿真模型:电压电流双闭环控制,直流输入电压范围广泛,输出交流峰值电压可调,高效频率控制技术在1-200hz之间 ,双极性SPWM单相全桥逆变电路仿真模型:电压电流双闭环控
- MATLAB程序实现:多无人船协同围捕控制算法-3船围捕运动船只仿真,距离监控与学习参考,MATLAB程序实现:多无人船协同围捕控制算法-3船围捕运动船只仿真与距离动态调整,MATLAB程序:多个
- QQPinyin QQ拼音输入法安装包版本,主要用于在Windows系统上安装和使用QQ拼音输入法
- log4cplus-2.0.8动态库,静态库
- FX3U定位与气缸FB块直接调用修改范例:新颖写法展现清晰思路,FB块学习宝典 ,FX3U定位与气缸FB块编程范例:开放访问,新颖写法展现清晰思路,FX3U的定位和气缸的FB块,没有密码,可以随便直接
- 2018年世界排名前50的餐厅.zip
- 六电池智能均衡系统:高精度、快速均衡的Buck-Boost电路解决方案,高效电池均衡技术:快速精准调节BuckBoost电路,提升电池性能与寿命,6个电池均衡,buckboost电路,精度高,均衡速度
- 零基础入门转录组下游分析-机器学习算法之boruta(训练模型)教程配套资源
- springboot091创新创业教育中心项目申报管理系统a_zip.zip
- 智能同步控制程序:S7-200 Smart在卷板材生产线与造纸设备的应用,多机同步控制与速度频率同步程序-专为卷板材生产线和造纸设备设计,速度同步频率同步程序 s7-200smart程序 适合卷板材
- 2021年世界幸福指数.zip
- file-download sftp
- MATLAB环境下多领域应用的高效变分模态分解算法优化研究:涵盖金融时间序列、地震微震信号、机械振动等多类信号的处理,MATLAB环境下多领域应用的高效变分模态分解算法优化研究:涵盖金融时间序列、地震
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


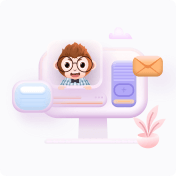
- 1
- 2
- 3
- 4
- 5
- 6
前往页