package com.atguigu.lease.web.admin.service.impl;
import com.atguigu.lease.common.exception.LeaseException;
import com.atguigu.lease.common.result.ResultCodeEnum;
import com.atguigu.lease.model.entity.*;
import com.atguigu.lease.model.enums.ItemType;
import com.atguigu.lease.model.enums.LeaseStatus;
import com.atguigu.lease.web.admin.mapper.RoomInfoMapper;
import com.atguigu.lease.web.admin.service.*;
import com.atguigu.lease.web.admin.vo.attr.AttrValueVo;
import com.atguigu.lease.web.admin.vo.graph.GraphVo;
import com.atguigu.lease.web.admin.vo.room.RoomDetailVo;
import com.atguigu.lease.web.admin.vo.room.RoomItemVo;
import com.atguigu.lease.web.admin.vo.room.RoomQueryVo;
import com.atguigu.lease.web.admin.vo.room.RoomSubmitVo;
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper;
import com.baomidou.mybatisplus.extension.plugins.pagination.Page;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import lombok.extern.slf4j.Slf4j;
import org.springframework.beans.BeanUtils;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.context.annotation.Lazy;
import org.springframework.stereotype.Service;
import org.springframework.util.CollectionUtils;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Logger;
/**
* @author liubo
* @description 针对表【room_info(房间信息表)】的数据库操作Service实现
* @createDate 2023-07-24 15:48:00
*/
@Service
public class RoomInfoServiceImpl extends ServiceImpl<RoomInfoMapper, RoomInfo>
implements RoomInfoService {
@Autowired
private GraphInfoService graphInfoService;
@Autowired
private RoomAttrValueService roomAttrValueService;
@Autowired
private RoomFacilityService roomFacilityService;
@Autowired
private RoomLabelService roomLabelService;
@Autowired
private RoomPaymentTypeService roomPaymentTypeService;
@Autowired
private RoomLeaseTermService roomLeaseTermService;
@Lazy
@Autowired
private LeaseAgreementService leaseAgreementService;
@Autowired
private RoomInfoMapper roomInfoMapper;
@Lazy
@Autowired
private ApartmentInfoService apartmentInfoService;
@Autowired
private FacilityInfoService facilityInfoService;
@Autowired
private LabelInfoService labelInfoService;
/**
* @Description: 先看看lease_agreement里面有没有租约(租约状态是2 , 5),没有租约再删除房间
* @Param: [id]
* @return: void
* @Author: simonf
* @Date: 2024/1/24
*/
@Override
public void customRemoveRoomById(Long id) {
//先看看lease_agreement里面有没有租约(租约状态是2,5)
// 1.看看房间里面有没有租约,(多表关联查询,根据传入的id查询Lease_Agreement表里的room_id)
LambdaQueryWrapper<LeaseAgreement> leaseAgreementIfNull = new LambdaQueryWrapper<>();
leaseAgreementIfNull.eq(LeaseAgreement::getRoomId, id);
Long count = leaseAgreementService.count(leaseAgreementIfNull);
if (count > 0) {
// 2.看看租约状态
LambdaQueryWrapper<LeaseAgreement> leaseAgreementQueryWrapper = new LambdaQueryWrapper<>();
leaseAgreementQueryWrapper.in(LeaseAgreement::getStatus, LeaseStatus.SIGNED,LeaseStatus.WITHDRAWING);
List<LeaseAgreement> list = leaseAgreementService.list(leaseAgreementQueryWrapper);
//3.如果租约还有且状态为(2,5)抛异常
System.out.println(list+"这是租约相关对象");
if (!list.isEmpty()) {
throw new LeaseException(ResultCodeEnum.DELETE_ERROR);
}
}
//1.删除RoomInfo
super.removeById(id);
//2.删除graphInfoList
LambdaQueryWrapper<GraphInfo> graphQueryWrapper = new LambdaQueryWrapper<>();
graphQueryWrapper.eq(GraphInfo::getItemType, ItemType.ROOM);
graphQueryWrapper.eq(GraphInfo::getItemId, id);
graphInfoService.remove(graphQueryWrapper);
//3.删除attrValueList
LambdaQueryWrapper<RoomAttrValue> attrQueryWrapper = new LambdaQueryWrapper<>();
attrQueryWrapper.eq(RoomAttrValue::getRoomId, id);
roomAttrValueService.remove(attrQueryWrapper);
//4.删除facilityInfoList
LambdaQueryWrapper<RoomFacility> facilityQueryWrapper = new LambdaQueryWrapper<>();
facilityQueryWrapper.eq(RoomFacility::getRoomId, id);
roomFacilityService.remove(facilityQueryWrapper);
//5.删除labelInfoList
LambdaQueryWrapper<RoomLabel> labelQueryWrapper = new LambdaQueryWrapper<>();
labelQueryWrapper.eq(RoomLabel::getRoomId, id);
roomLabelService.remove(labelQueryWrapper);
//6.删除paymentTypeList
LambdaQueryWrapper<RoomPaymentType> paymentQueryWrapper = new LambdaQueryWrapper<>();
paymentQueryWrapper.eq(RoomPaymentType::getRoomId, id);
roomPaymentTypeService.remove(paymentQueryWrapper);
//7.删除leaseTermList
LambdaQueryWrapper<RoomLeaseTerm> termQueryWrapper = new LambdaQueryWrapper<>();
termQueryWrapper.eq(RoomLeaseTerm::getRoomId, id);
roomLeaseTermService.remove(termQueryWrapper);
}
@Override
public void saveOrUpdateRoom(RoomSubmitVo roomSubmitVo) {
boolean isUpdate = roomSubmitVo.getId() != null;
super.saveOrUpdate(roomSubmitVo);
//若为更新操作,则先删除与Room相关的各项信息列表
if (isUpdate) {
//1.删除原有graphInfoList
LambdaQueryWrapper<GraphInfo> graphQueryWrapper = new LambdaQueryWrapper<>();
graphQueryWrapper.eq(GraphInfo::getItemType, ItemType.ROOM);
graphQueryWrapper.eq(GraphInfo::getItemId, roomSubmitVo.getId());
graphInfoService.remove(graphQueryWrapper);
//2.删除原有roomAttrValueList
LambdaQueryWrapper<RoomAttrValue> attrQueryMapper = new LambdaQueryWrapper<>();
attrQueryMapper.eq(RoomAttrValue::getRoomId, roomSubmitVo.getId());
roomAttrValueService.remove(attrQueryMapper);
//3.删除原有roomFacilityList
LambdaQueryWrapper<RoomFacility> facilityQueryWrapper = new LambdaQueryWrapper<>();
facilityQueryWrapper.eq(RoomFacility::getRoomId, roomSubmitVo.getId());
roomFacilityService.remove(facilityQueryWrapper);
//4.删除原有roomLabelList
LambdaQueryWrapper<RoomLabel> labelQueryWrapper = new LambdaQueryWrapper<>();
labelQueryWrapper.eq(RoomLabel::getRoomId, roomSubmitVo.getId());
roomLabelService.remove(labelQueryWrapper);
//5.删除原有paymentTypeList
LambdaQueryWrapper<RoomPaymentType> paymentQueryWrapper = new LambdaQueryWrapper<>();
paymentQueryWrapper.eq(RoomPaymentType::getRoomId, roomSubmitVo.getId());
roomPaymentTypeService.remove(paymentQueryWrapper);
//6.删除原有leaseTermList
LambdaQueryWrapper<RoomLeaseTerm> termQueryWrapper = new LambdaQueryWrapper<>();
termQueryWrapper.eq(RoomLeaseTerm::getRoomId, roomSubmitVo.getId());
roomLeaseTermService.remove(termQueryWrapper);
}
//1.保存新的graphInfoList
List<GraphVo> graphVoList = roomSubmitVo.getGraphVoList();
if (!CollectionUtils.isEmpty(graphVoList)) {
ArrayList<GraphInfo> graphInfoList = new ArrayList<>();
for (GraphVo graphVo : graphVoList) {
GraphInfo graphInfo = new GraphInfo();
graphInfo.setItemType(ItemType.ROOM);
graphInfo.setItemId(roomSubmitVo.getId());
graphInfo.setName(graphVo.getName());
graphInfo.setUrl(graphVo.getUrl());
graphInfoList.add(graphInfo);
}
graphInfoService.saveBatch(graphInfoList);
}
//2.保存新的
没有合适的资源?快使用搜索试试~ 我知道了~
温馨提示
尚庭公寓租赁平台 - 基于Java开发,包含367个文件,如JAVA、XML、YML、LICENSE、MD和GITIGNORE等。该平台为用户提供找房、看房预约、租约管理等功能,同时为管理员提供公寓(房源)管理、租赁管理、用户管理等功能,是一个完整的公寓租赁解决方案。
资源推荐
资源详情
资源评论
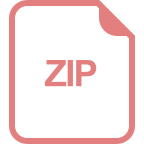
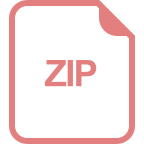
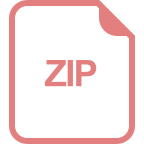
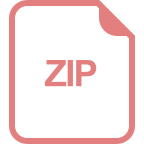
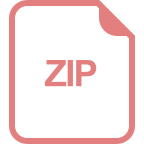
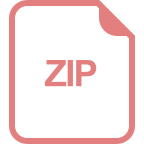
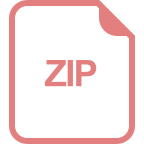
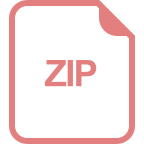
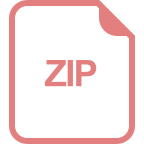
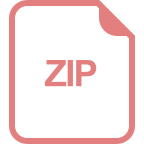
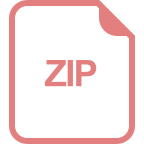
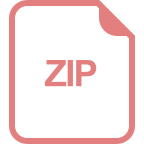
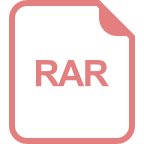
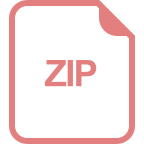
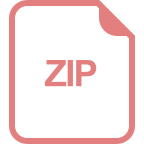
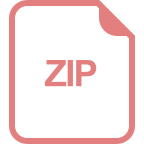
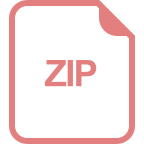
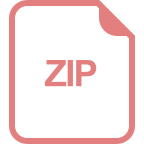
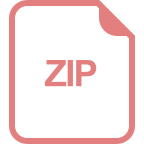
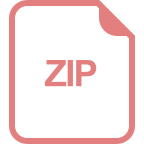
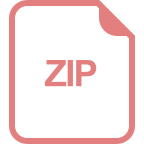
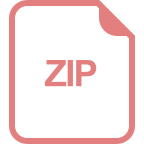
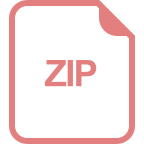
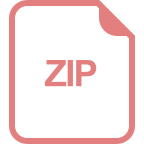
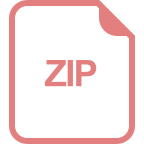
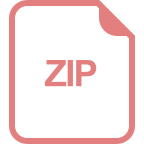
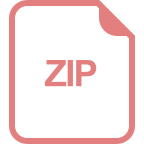
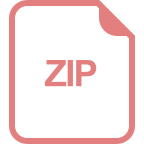
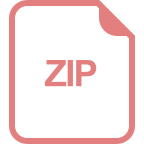
收起资源包目录

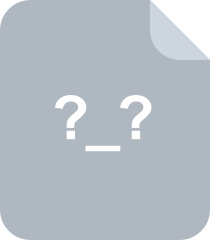
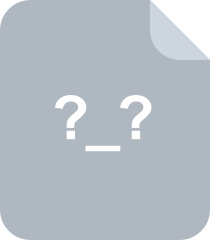
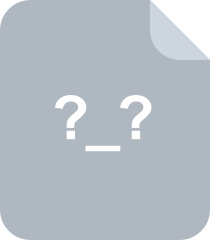
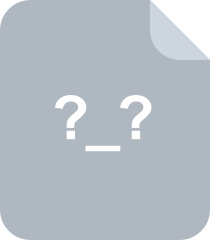
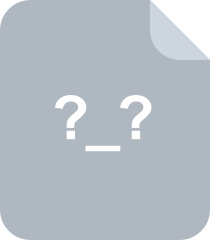
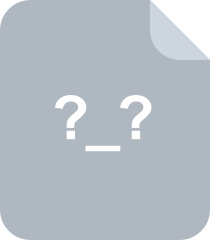
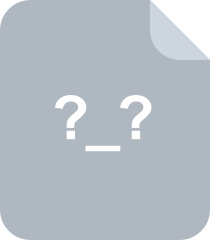
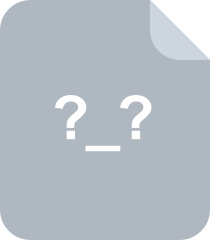
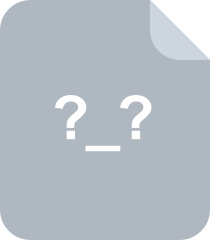
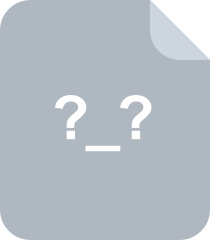
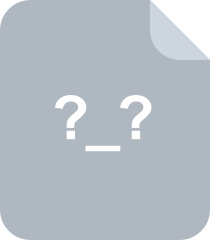
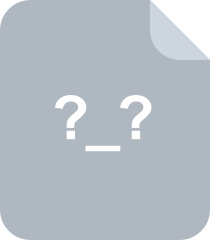
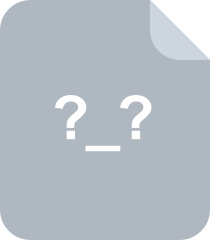
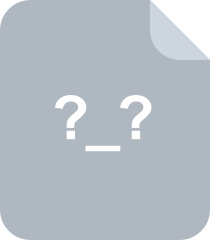
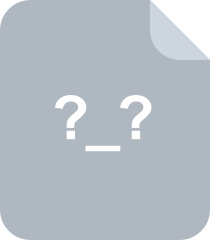
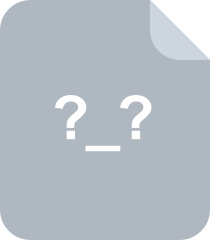
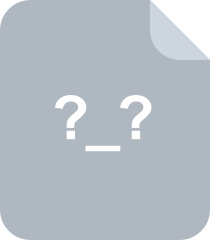
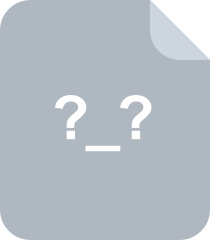
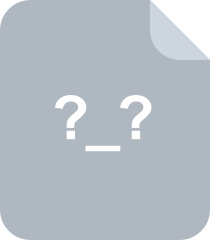
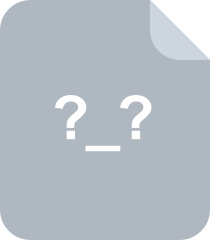
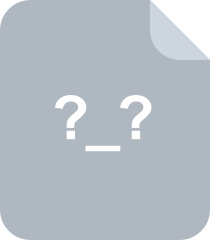
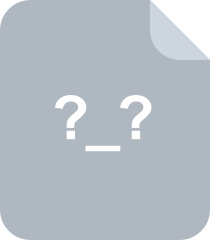
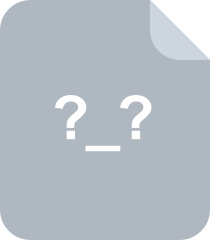
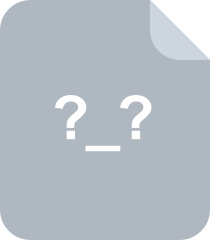
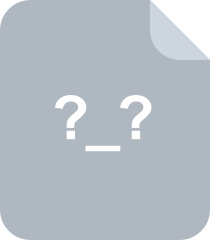
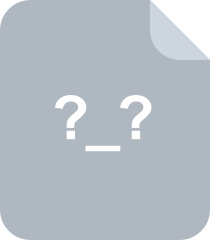
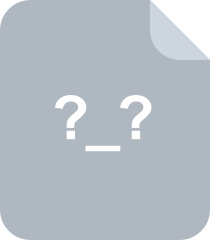
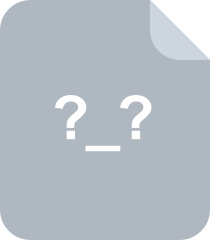
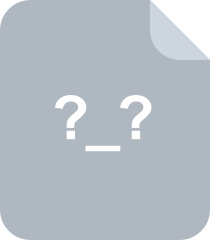
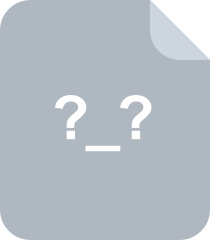
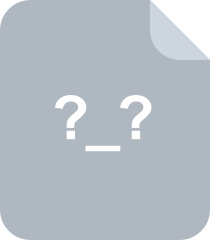
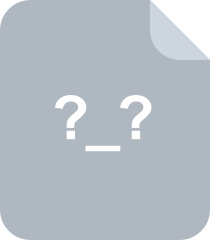
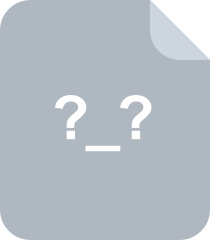
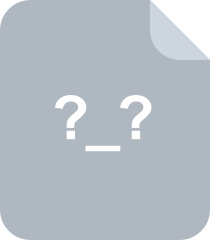
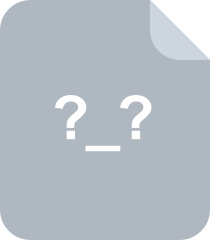
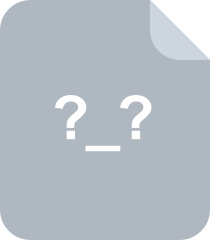
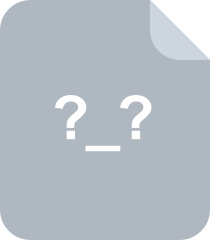
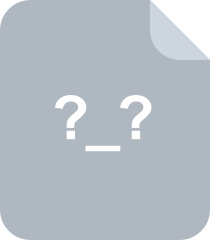
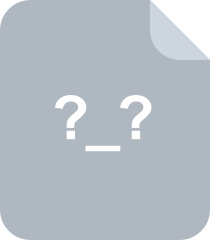
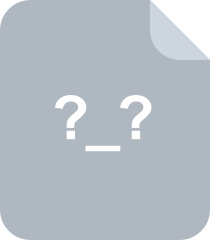
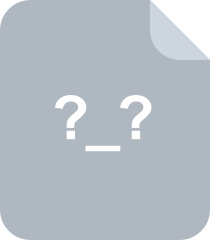
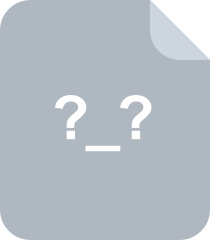
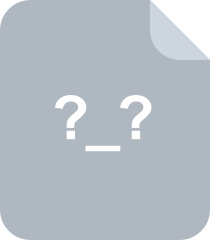
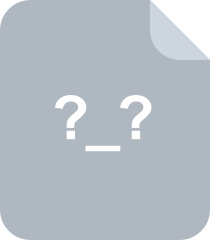
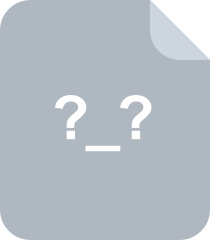
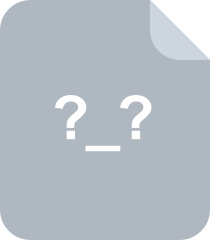
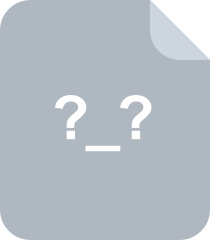
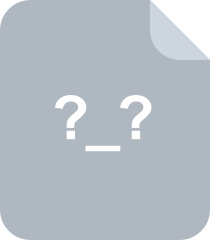
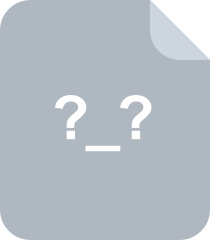
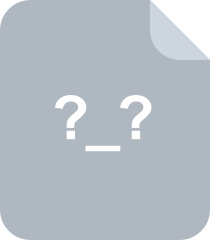
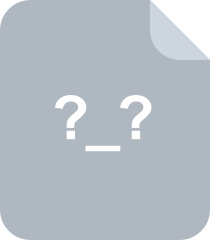
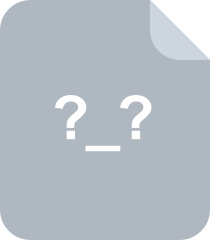
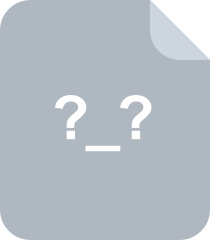
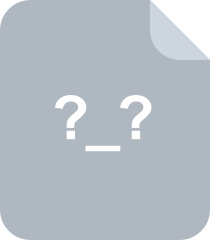
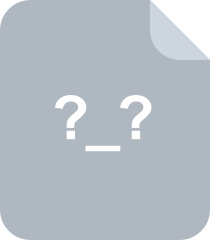
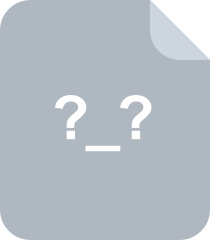
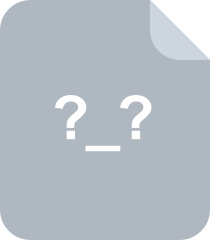
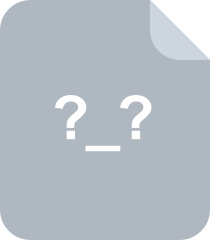
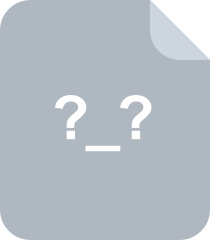
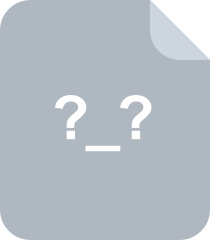
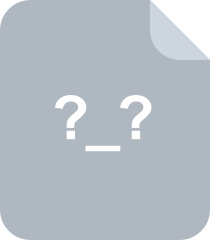
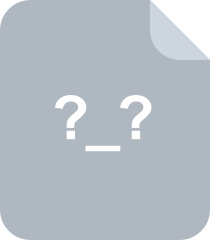
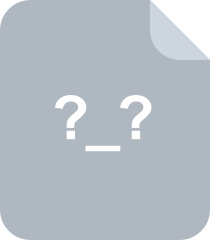
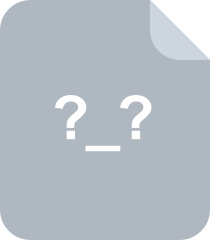
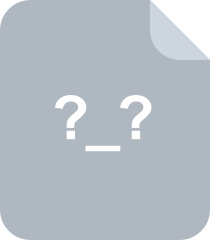
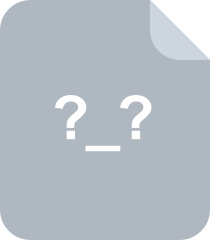
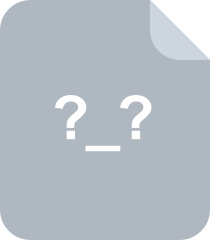
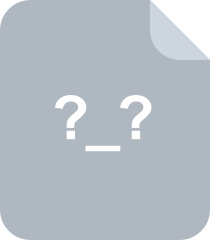
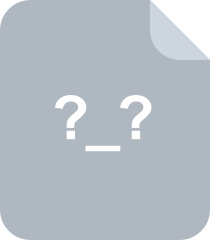
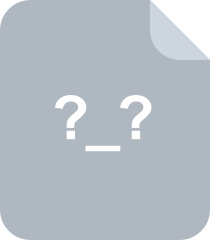
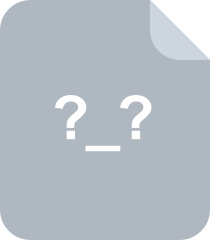
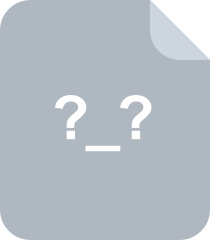
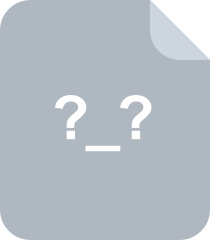
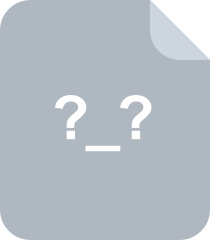
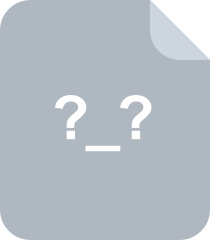
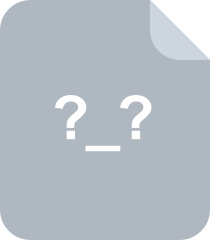
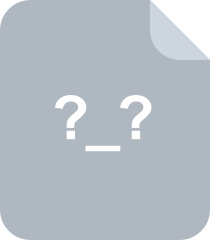
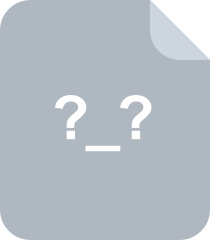
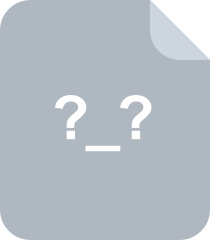
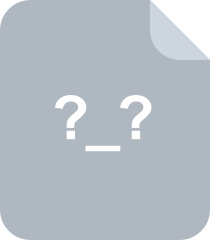
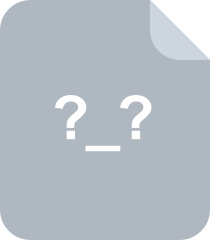
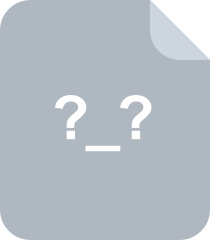
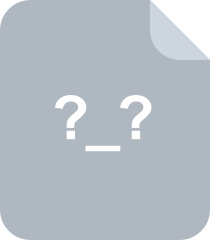
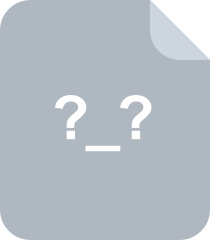
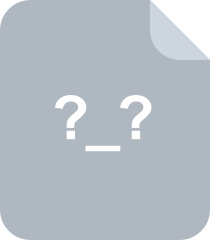
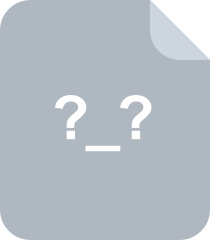
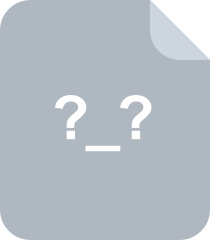
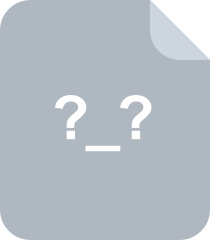
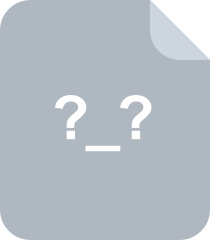
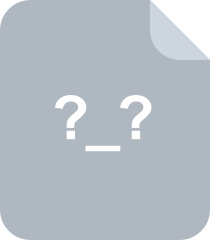
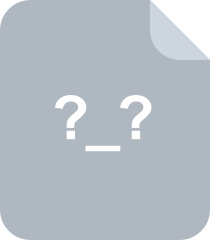
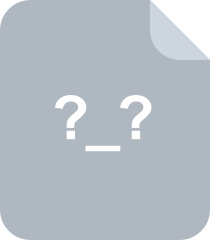
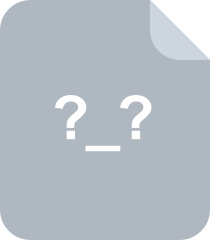
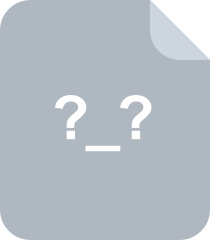
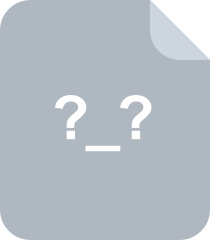
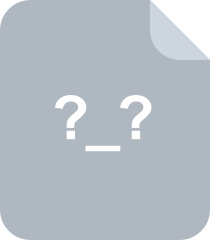
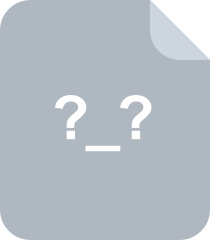
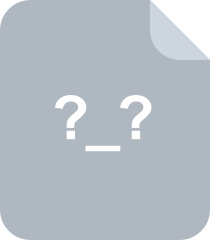
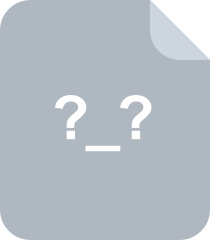
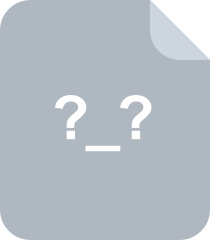
共 367 条
- 1
- 2
- 3
- 4
资源评论
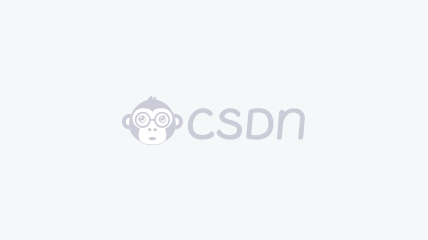

沐知全栈开发
- 粉丝: 5703
- 资源: 5219
上传资源 快速赚钱
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

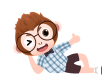
最新资源
资源上传下载、课程学习等过程中有任何疑问或建议,欢迎提出宝贵意见哦~我们会及时处理!
点击此处反馈


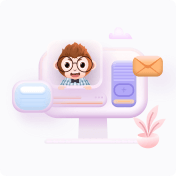
安全验证
文档复制为VIP权益,开通VIP直接复制
